EuroPython 2015 - Day One
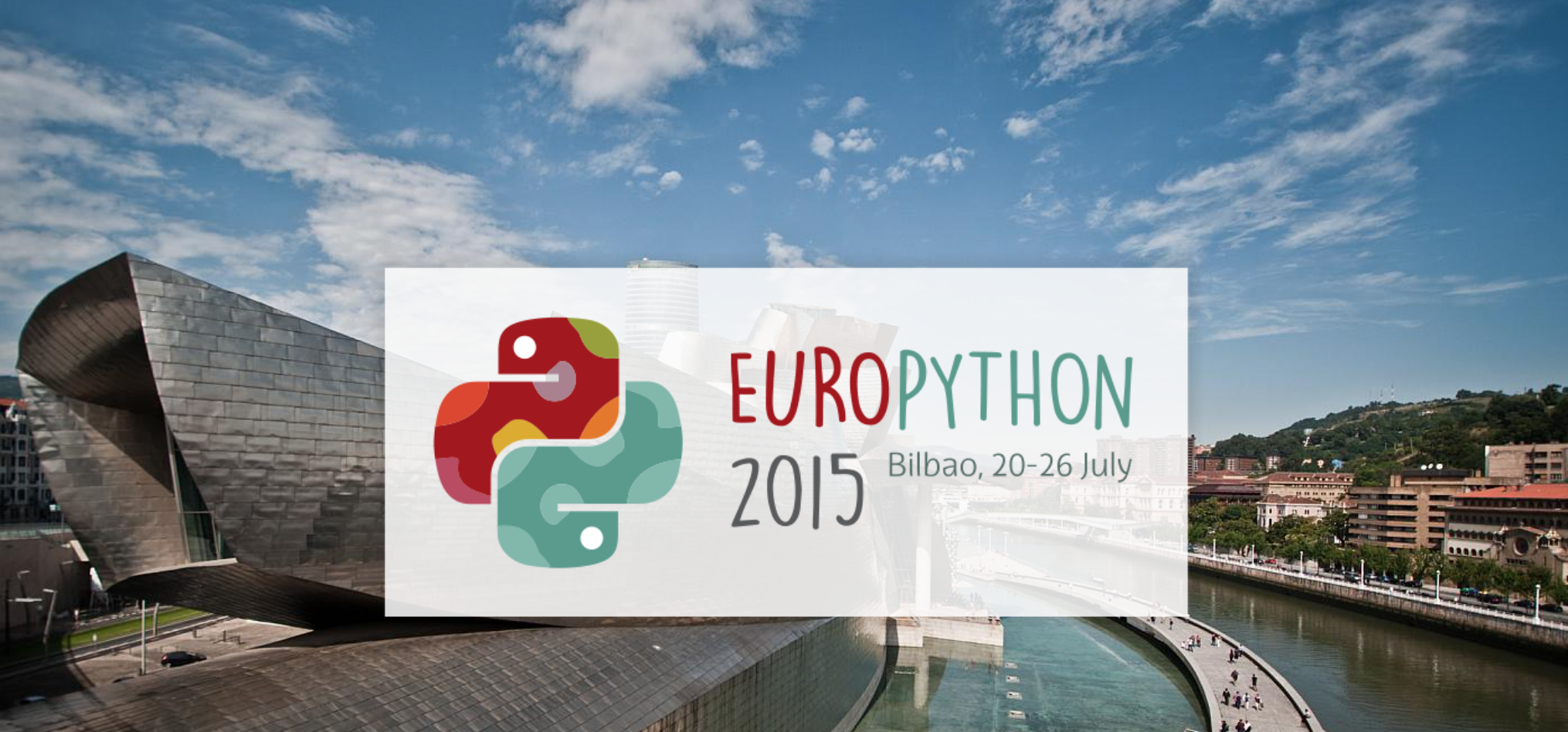
Below are my notes, they might be wrong, please check the actual coverage.
Djangogirls - Keynote
Ola & Ola from the DjangoGirls foundation are writing a book called yay python.
Asyncio
We learned how easy it is do asynchronous programming in Python 3.4+ using asyncio.
Check out the aiohttp documentation.
The following is a quick untested example.
1 | pip install asyncio aiohttp |
1 | import asyncio |
You can add routes like this:
1 | app.router.add_route( ... ) |
The libary support is quite nice at the moment, you can see
them all here at asyncio.org.
Featuring:
- aiopg
- aiozmq
- asyncio-redis
- aiomemcache
Container based Linux flavours
Presenter: @hguemar
Presenting and overview of containerization OS’s.
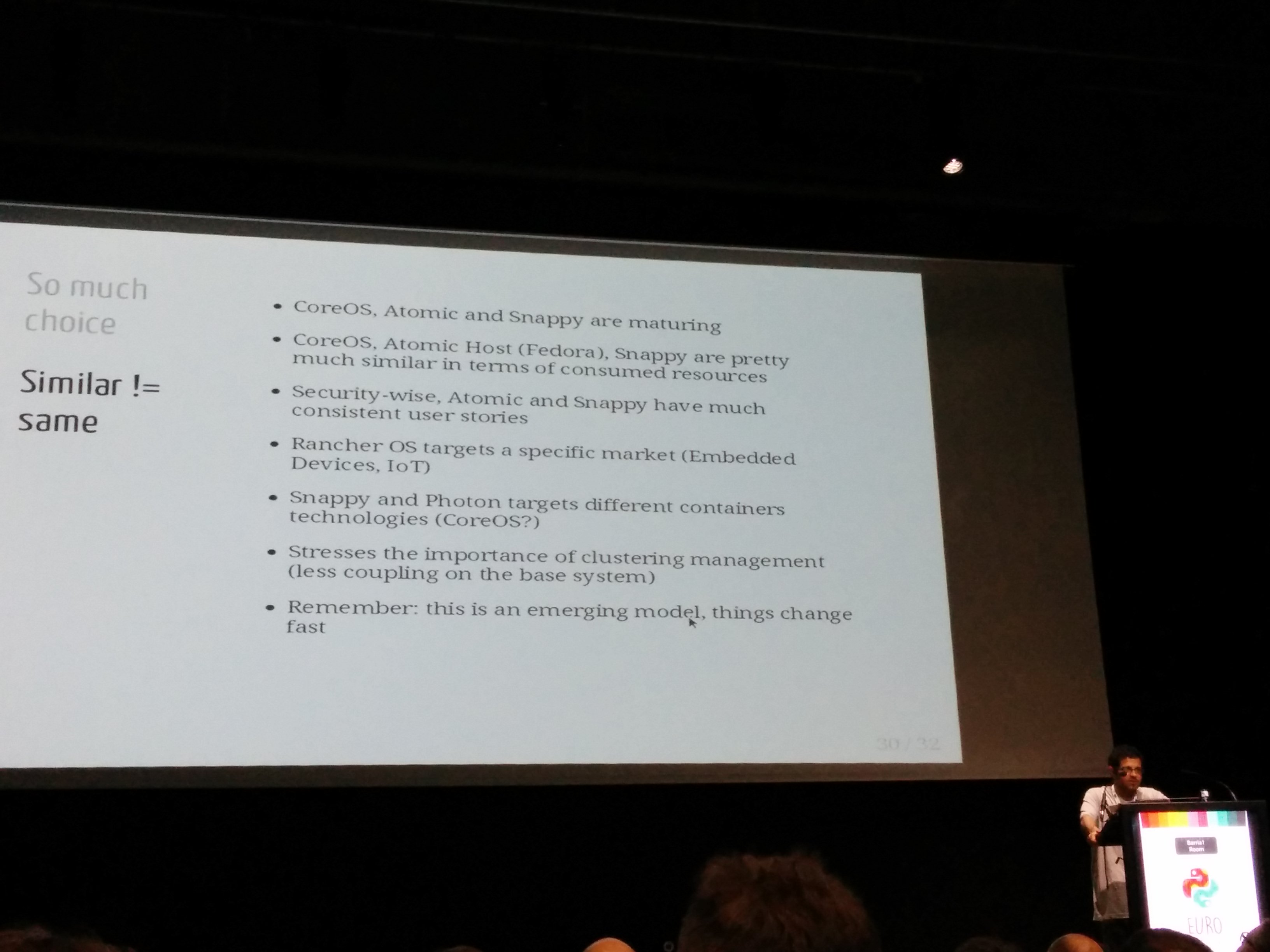
All of the OS’s share the following (mostly):
- SystemD
- etcd
- cloud-init
- kubernets
The OS’s
- CoreOS
- based on ChromiumOS that is itself based on Gentoo
- requires a running of a toolbox container which is based on Fedora
- Project Atomic by Redhat
- not yum but rpm-ostree (from the GNOME CI)
- SELinux secured containers by Dan Walsh
- Snappy Ubuntu
- uses AppArmor and LXD
- based on Canonicals work on phones and JeOS
- Photon by VMWare
- based on Fedora
- uses rpm-ostree and a yum compatible tdnf
- not production ready yet (July 2015)
- Rancher OS
- radically different
- very minimal footprint (~20MB)
- runs a Docker instance as PID1
- doesn’t use SystemD, but instead
- uses a System container and a user container
- basically docker inception
- targets Embedded Devices, IoT
A generic API wrapper
Presenter: @xima
Made a universal API wrapper library called tapioca. github.
Tries to make it easy to wrap RESTful http API’s and binds them in a pythonic way.
Does pagination.
Currently supports:
- Facebook,
- Twitter,
- Parse.com
- Mandrill
Combining Rust and Python
Dimitry Trofaimov wanted to try Rust and used it to build a Python profiler.
Rust started 2010 - now version 1.x since May 2015.
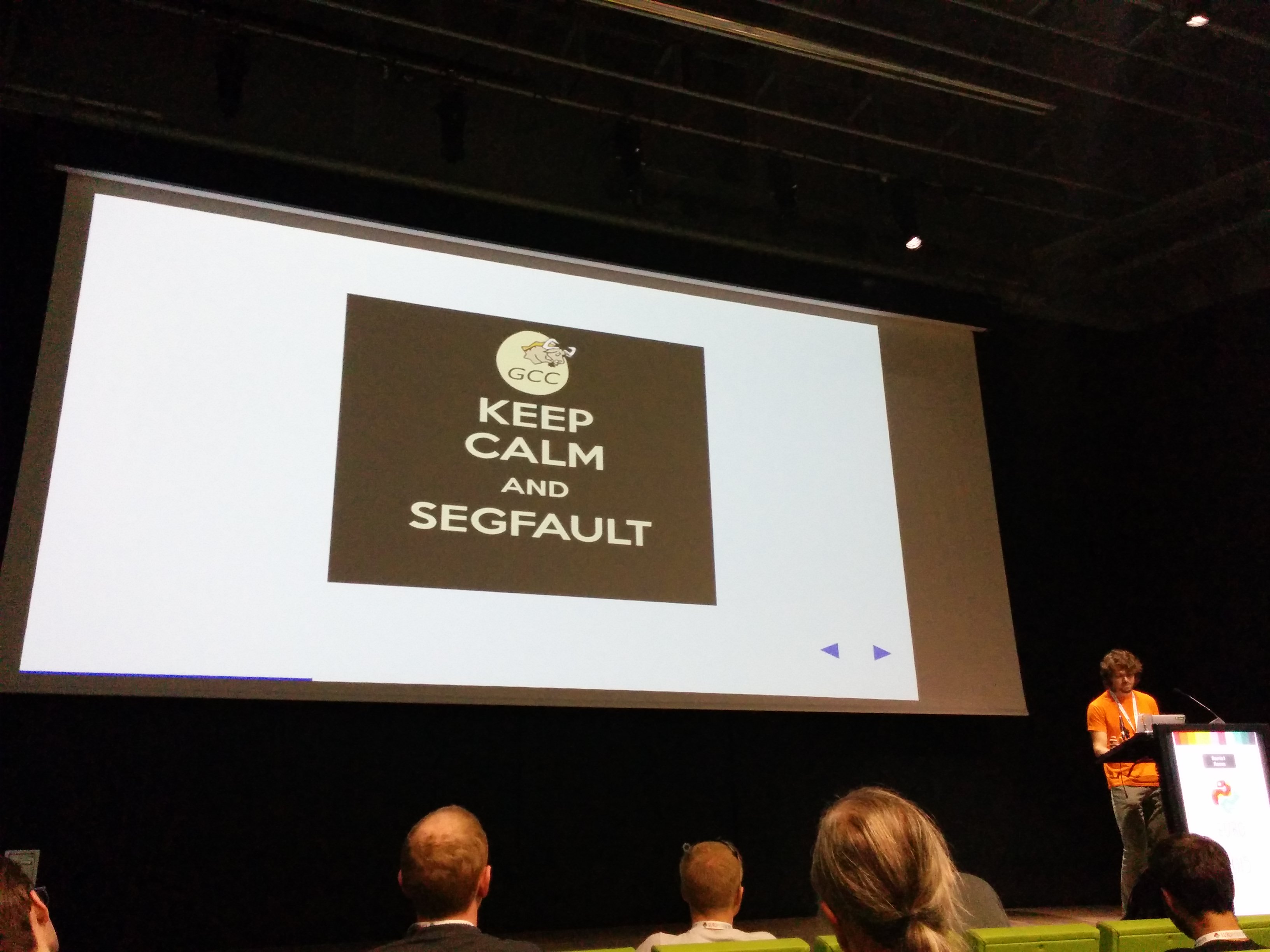
Now, normally when you search how to integrate Rust with Python, you’ll come across
FFI. However, for a profiler this isn’t enough.
When creating a Python profiler there are two options:
- tracing
- statistical (periodically captures frames of function stacks)
Datatypes aren’t easily exchangable between Python and Rust, fortunately,
there is a nice library mio github.
1 | extern crate cpython; |